We use string interpolation to embed expressions or dynamic data from our components in the HTML markup. A {{ }}
is used as the delimiter for interpolation.
Let’s see this in action. Open app.component.ts and replace its content with this code.
<h1>{{ 1+2 }}</h1>
Here, we’re binding an expression in our HTML markup. When we run our application, Angular will evaluate the expression and bind its result in the <h1>
tag. Run ng serve
to see the output.
Binding properties of a component
With interpolation, we can bind the values or properties of a component in HTML markup. Look at this example.
app.component.ts
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
title = 'LearnAngular';
}
Here we have a property named title in the component. We can render this value in the HTML code.
app.component.html
<h1>{{ title }}</h1>
Angular updates the view
Angular will automatically update the view if the value of the property rendered in a view changes. This is one of the most beautiful feature of Angular. Let’s try to understand this with an example. In this code I created a constructor in the app component class that continuously updates the time property after waiting for one second.
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
time:number = 0;
constructor(){
setInterval(() => {
this.time += 1;
}, 1000);
}
}
And in the HTML markup:
<h1>{{ time + " Seconds(s) "}}</h1>
Here’s the output
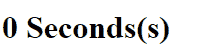
Points to remember
- Interpolation is used to embed expression or dynamic data from a component.
- We use
{{ }}
as the delimiter for interpolation. - Angular will automatically update the view if the value a property changes.
In the next post, we’ll lean about pipes.